Using Selenium Testing for Electron (Atom shell) Applications
Electron (formerly Atom Shell) is a very new way to quickly create javascript applications for multiple platforms. Not a lot of documentation exists about using Selenium to do full-fledged integration testing on Electron Applications. And even less exists about performing such tests in python. This brief tutorial should answer a few questions about Electron Applications and show you how to get started using Selenium to test them.
2) desired_capabilities: dictionary specifying location of Electron App executable
** Use chromeOptions since using an Electron app requires Chrome
** On OSX binary is inside ".app" directory, for me its: "Electron.app/Contents/MacOS/Electron"
3) browser_profile: A special setting used only for Firefox
4) proxy: If your application/chromedriver is not running locally
5) keep_alive: Leave as False
To access the buttons on our GUI make this call from a python script/interpreter:
You can then access the buttons on you application through the 'buttons' object in the python script. You can click, view button text, and even edit DOM attributes all through Selenium. To access the "Linked Token File" button and call its associated action I can do this.
2) Executing Remote Scripts
- remote.execute_script("var scope = angular.element(document.getElementsByTagName('button')).scope(); scope.$apply(function(){scope.openMetadataForm()})") - remote.execute_script("var scope = angular.element(document.getElementsByClassName('form-group')).scope(); scope.$apply(scope.metadata.fileType='BAM')") 3) Executing Remote Scripts (Asyncronously)
- Access specific Elements using selenium. - Our GUI is loaded with buttons and fields as well as nested inside of an angular application.
I. Getting started
Install and start chromedriver
Selenium need this to be able to make calls to the Electron App. Chromedriver acts as a bridge between Selenium and Chrome, it follows Selenium wire protocol. By default, chromium runs on port 9515, you can start on alternate ports, but remember the assigned port this will be passed as an argument to Selenium later../chromedriver --port=9515
Install Selenium
You'll need to use Selenium's remotewebdriver to interface with chromedriver. Im using a python virtualenv to keep all my python plugins isolated from other apps.pip install selenium
Pick a testing framework
I am using python behave, which organizes code into feature files (readable outline of test cases) and implementation filespip install behave
II. Creating Tests
Starting Python Script
Using webdriver allows us to launch the Electron App through python code. Simply import webdriver and the follow lines should launch your application:from selenium import webdriver
remote = webdriver.remote.webdriver.WebDriver(command_executor='http://localhost:9515', desired_capabilities = {'chromeOptions':{ 'binary': '/Applications/Electron.app/Contents/MacOS/Electron'}}, browser_profile=None, proxy=None, keep_alive=False)
Arguments:
1) command_executor: Local or remote port where chromedriver is running2) desired_capabilities: dictionary specifying location of Electron App executable
** Use chromeOptions since using an Electron app requires Chrome
** On OSX binary is inside ".app" directory, for me its: "Electron.app/Contents/MacOS/Electron"
3) browser_profile: A special setting used only for Firefox
4) proxy: If your application/chromedriver is not running locally
5) keep_alive: Leave as False
Selenium Basics
After this you should see the first page of your Electron Application pop up! Selenium makes it easy to grab DOM elements and view their properties and even perform simple tasks such as clicking buttons. For example, the first page of my application is this: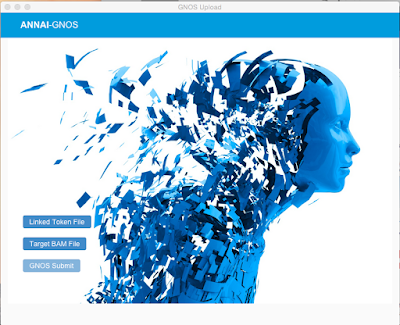
To access the buttons on our GUI make this call from a python script/interpreter:
buttons = remote.find_elements_by_tag_name("button")
You can then access the buttons on you application through the 'buttons' object in the python script. You can click, view button text, and even edit DOM attributes all through Selenium. To access the "Linked Token File" button and call its associated action I can do this.
buttons[0].text
buttons[0].click()
Tackling AngularJS
While Angular makes for organized code, the use of controllers also abstracts away some elements that would normally be easily manipulated through html. This means that simple javascript actions that could be called directly, require a small work-around. Because chromedriver can render all our client side javascript and even register callback methods that can really help to make selenium much more usable for Electron Apps.2) Executing Remote Scripts
remote.execute_script(script, args)
- remote.execute_script("var scope = angular.element(document.getElementsByTagName('button')).scope(); scope.$apply(function(){scope.openMetadataForm()})") - remote.execute_script("var scope = angular.element(document.getElementsByClassName('form-group')).scope(); scope.$apply(scope.metadata.fileType='BAM')") 3) Executing Remote Scripts (Asyncronously)
remote.execute_async_script(script, args)
- Access specific Elements using selenium. - Our GUI is loaded with buttons and fields as well as nested inside of an angular application.
Hello,
ReplyDeleteThe Article on Using Selenium Testing for Electron Applications is nice. It give detail information about it .Thanks for Sharing the information Selenium Testing. Software Testing Services
Can we control chrom browser + electron browser same time in single test case ?
ReplyDeleteusing switch browser command
Great tips :) I downloaded update version of chrome driver from Chrome Driver Please Read the Release note as well Here If chrome Browser is updated then you need to downloaded new chormedriver from the above link because it would be compact-able with new browser version. thank you~ Charlotte W. from offshore development team
ReplyDeleteSwiss replica uk watches, combining elegant style and cutting-edge technology, a variety of styles of Swiss replica uk a lange sohne watches, the pointer walks between your exclusive taste style.
ReplyDeleteThanks for a very interesting blog. What else may I get that kind of info written in such a perfect approach? I’ve a undertaking that I am simply now operating on, and I have been at the look out for such info. Tableau Data Blending
ReplyDeleteAwesome blog. I enjoyed reading your articles. This is truly a great read for me. I have bookmarked it and I am looking forward to reading new articles. Keep up the good work!
ReplyDeletearttificial intelligence course in lucknow
After reading your article I was amazed. I know that you explain it very well. And I hope that other readers will also experience how I feel after reading your article.
ReplyDeletearttificial intelligence course in patna
Awesome blog. I enjoyed reading your articles. This is truly a great read for me. I am looking forward to reading new articles. Keep up the good work! I would like to add a little comment data science training in hyderabad
ReplyDeleteExcellent Blog! I would like to thank for the efforts you have made in writing this post. I am hoping the same best work from you in the future as well. I wanted to thank you for this websites! Thanks for sharing. Great websites!
ReplyDeletebusiness analytics course in hyderabad
data science course hyderabad
data analytics courses in hyderabad
I’m going to read this. I’ll be sure to come back. thanks for sharing. and also This article gives the light in which we can observe the reality. this is very nice one and gives indepth information. thanks for this nice article... Outlook based CRM
ReplyDeleteI real delighted to find this site on bing, just what I was looking for : D also bookmarked . data science from scratch
ReplyDeleteMMORPG
ReplyDeleteİnstagram takipçi satın al
tiktok jeton hilesi
tiktok jeton hilesi
antalya saç ekimi
İnstagram takipçi satın al
INSTAGRAM TAKİPÇİ SATIN AL
metin2 pvp serverlar
instagram takipçi satın al
ReplyDeleteHey friend, it is very well written article, thank you for the valuable and useful information you provide in this post. Keep up the good work! n india
Thanks for sharing this valuable information,we provide youtube shorts downloader which helps to get more
ReplyDeleteideas to write more quality content.
This blog is a useful, up-to-date resource with interesting information. Check our IT Certification Course by SkillUp Online.
ReplyDelete